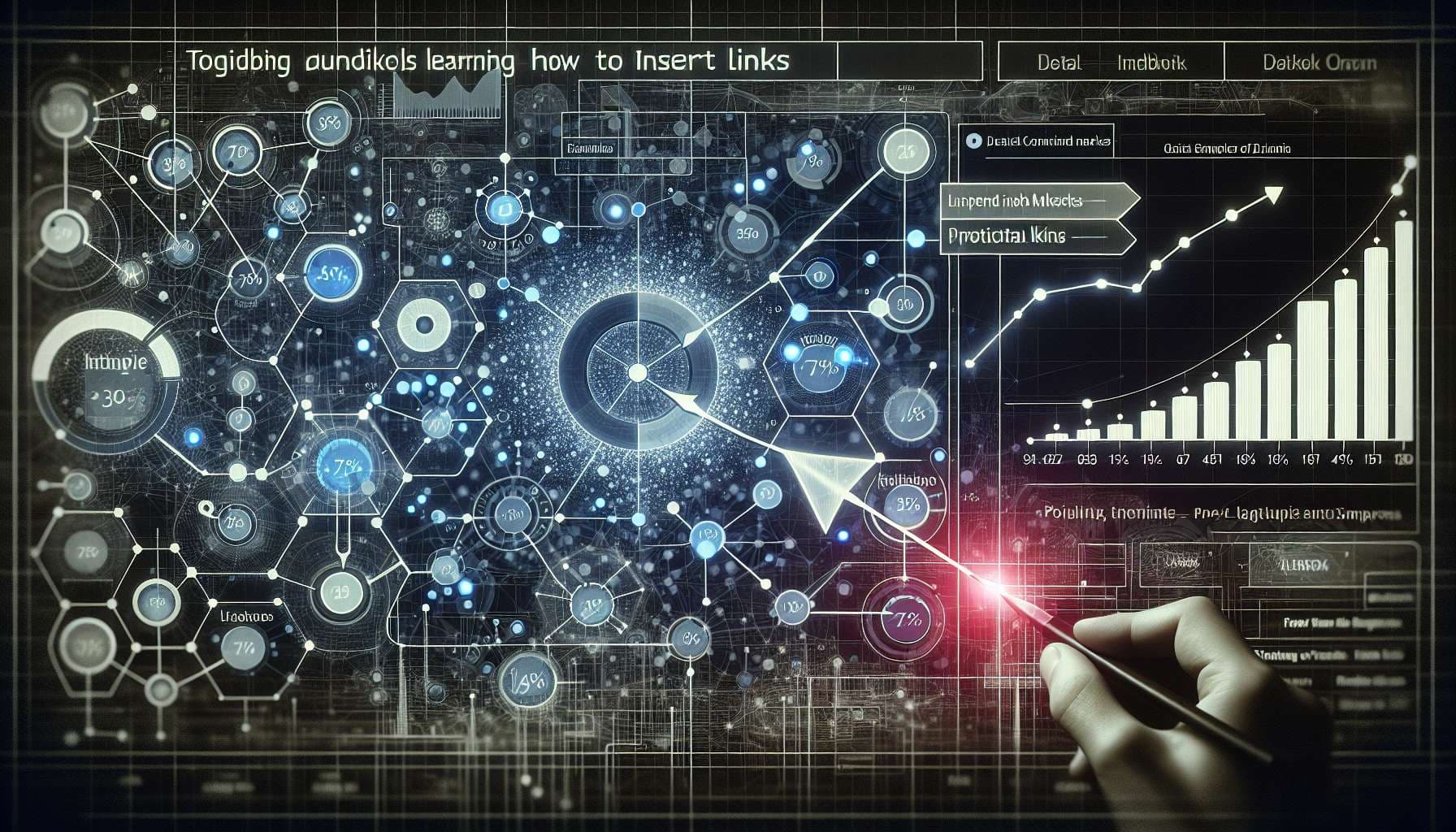
Here is an example of market — https://manifold.markets/RemNi/will-there-be-a-dyson-sphere-around-498a9441b3bf
You can see that there is very pretty list with links to other markets with percents.
Here is an image:
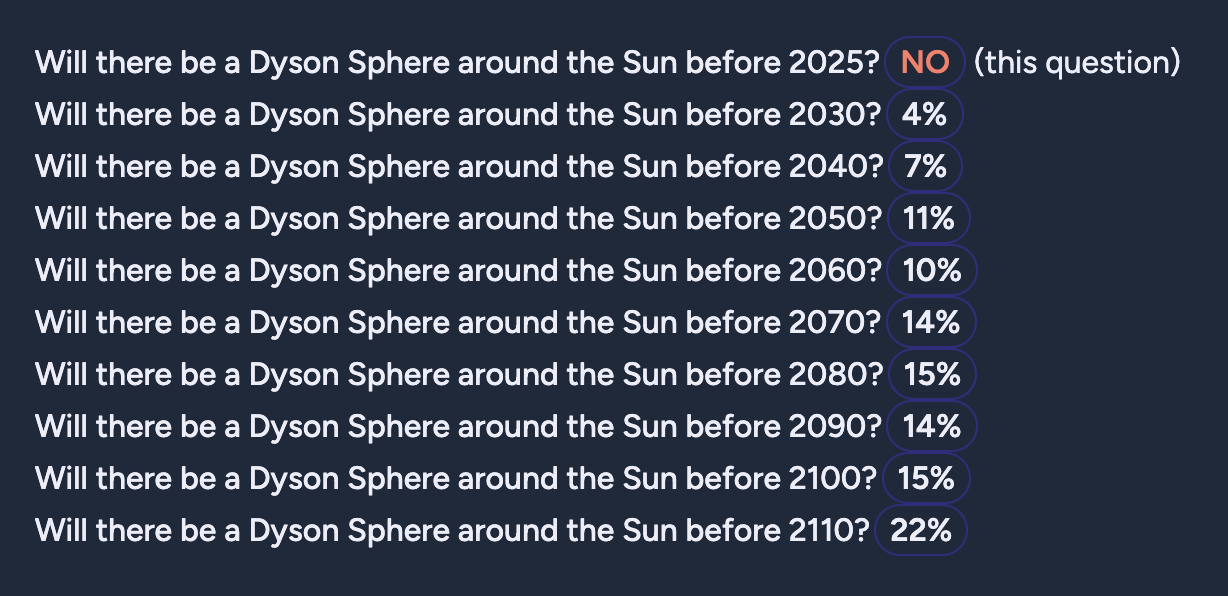
I want to find the way how I can set the same description via manifodl API.
Now I can change market description via:
url = f"https://api.manifold.markets/v0/market/{market_id}/update"
headers = {
'Authorization': f'Key {api_key}',
'Content-Type': 'application/json'
}
data = {
"question": title,
"descriptionMarkdown": body,
}
But I don't see how can i specify the links to the markets to be rendered as percent or resolution result.
About a year ago I was told how to do it via web interface — https://manifold.markets/bessarabov/teach-me-how-to-insert-links-to-mar
(but it looks like that now I can't add the closed market using this technic )
Now I'm searching for the way how to do it via API.
I'm going to give 1000 bounty to the person who explain me how i can do it.
I just did it successfully with:
import requests
import json
# --------------------------
# 1) CONFIGURE THESE VALUES
# --------------------------
API_KEY = "-"
# The slug of the market you want to mention
REF_SLUG = "will-sp-500-increase-in-2026"
# This label is the “display text” Manifold will show. Usually "username/slug"
LABEL = "bessarabov/will-sp-500-increase-in-2026"
# The ID of the market YOU want to update
TARGET_MARKET_ID = "uSntnSqhSL"
# A new or existing question/title for your market (optional)
NEW_QUESTION = "What will be the responsibilities of a web dev in 2030?"
# --------------------------
# 2) FETCH THE REFERENCE MARKET’S ID
# --------------------------
def get_market_id_by_slug(slug):
url = f"https://api.manifold.markets/v0/slug/{slug}"
r = requests.get(url)
if r.status_code != 200:
raise ValueError(f"Could not find market with slug '{slug}'. Response: {r.text}")
data = r.json()
return data["id"]
try:
ref_contract_id = get_market_id_by_slug(REF_SLUG)
print(f"Referenced market ID: {ref_contract_id}")
except ValueError as e:
print("ERROR:", e)
exit(1)
# --------------------------
# 3) BUILD THE TIPTAP JSON DOC
# --------------------------
tiptap_doc = {
"type": "doc",
"content": [
{
"type": "paragraph",
"content": [
{
"type": "contract-mention",
"attrs": {
# The internal ID of the contract you are mentioning
"id": ref_contract_id,
# The “label” can be the slug or any descriptive string
"label": LABEL
}
},
{
"type": "text",
"text": " <— This is a fancy pill link referencing that market!"
}
]
},
{
"type": "paragraph",
"content": [
{
"type": "text",
"text": "Additional text can go here..."
}
]
}
]
}
description_json_str = json.dumps(tiptap_doc)
# --------------------------
# 4) UPDATE YOUR MARKET’S DESCRIPTION
# --------------------------
def update_market_description(market_id, api_key, question, description_json):
url = f"https://api.manifold.markets/v0/market/{market_id}/update"
headers = {
"Authorization": f"Key {api_key}",
"Content-Type": "application/json",
}
data = {
"question": question,
# If you only want to specify Tiptap JSON, do not include descriptionMarkdown
"descriptionJson": description_json
}
resp = requests.post(url, headers=headers, json=data)
if resp.status_code == 200:
print("Market updated successfully!")
else:
print("Failed to update market.")
print("Status code:", resp.status_code)
print("Response:", resp.text)
update_market_description(
TARGET_MARKET_ID,
API_KEY,
NEW_QUESTION,
description_json_str,
)